What is Singleton Design Pattern?
The Singleton design pattern is –
- A Creational Design Pattern
- Used for resource-intensive objects
- Restricts multiple instantiations of a class and ensures that only one instance of the class exists in the JVM
- Provides a global access point to get the instance of the class
- Some common uses are logger objects, driver objects, caching, thread pools, database connections, etc.
Typical implementation
- Private constructor
- Private static variable
- Public static method [Global access point]
Challenges and Resolution for Singleton Objects
- Break By Reflection challenge and it’s fix
- Serialization & Deserialization issue and its fix
- Cloning Problem & its solution
- Multithreaded Environment Problem and Solution
- Multiple ClassLoader problem & Fix
- Other Robust ways to create singleton
- Garbage Collection issue and fix
Break by Reflection Problem
Break by Reflection Problem – Fixed
Serialization & Deserialization issue
Serialization & Deserialization issue – Fixed
Cloning Problem in Singleton
Cloning Problem in Singleton – Fixed
Multithreaded environment problem
Multithreaded environment problem – Fix
Multiple Classloader problems & Fix
Other robust ways to create a singleton
Static Holder Pattern
Singleton using Enum
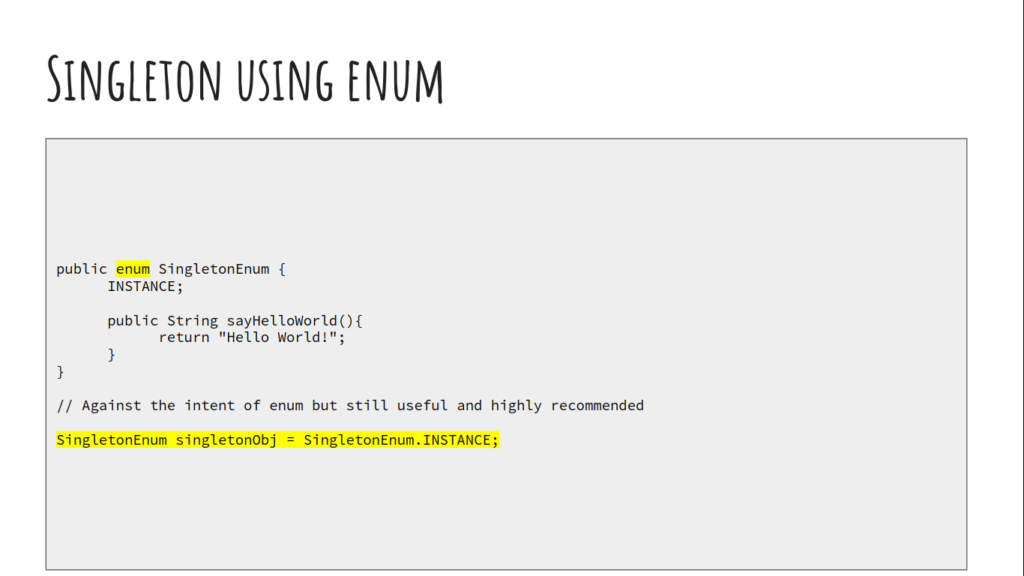
Check out This StackOverflow link for more descriptive discussion!
Garbage Collection issue and fix
Here is the GitHub link with the example code – https://github.com/webfuse2017/design-pattern-demo
Feel free to share your thoughts on this topic in the comments section below 👇 We would be happy to hear and discuss the same 🙂
2 thoughts on “Singleton Design Pattern: A Simple Way to Create Singleton Objects”
Really nice article on singleton design pattern. Explained very well!
Two Singletons will be created if the constructor runs and simultaneously another thread calls the method. Thread-safe code is particularly important in Singletons, since that Design Pattern is meant to give the user a single point of access that hides the complexities of the implementation, including multithreading issues.