Arrays in Java are a data structure that stores a collection of elements of the same data type. The elements of an array are stored in contiguous memory locations, which means that they are stored next to each other in memory. This makes it easy to access the elements of an array by their index.
How to declare an array in Java?
To declare an array in Java, you use the following syntax:
type[] arrayName = new type[size];
For example, to declare an array of integers, you would use the following code:
int[] numbers = new int[10];
This code creates an array of integers called numbers
with a size of 10.
How to access the elements of an array in Java?
You can access the elements of an array in Java by their index. The index of the first element in an array is 0, and the index of the last element is size - 1
.
For example, to access the first element of the numbers
array, you would use the following code:
int firstNumber = numbers[0];
This code will assign the value of the first element in the numbers
array to the variable firstNumber
.
How to iterate through an array in Java?
You can iterate through an array in Java using a for loop. The following code iterates through the numbers
array and prints the value of each element:
for (int i = 0; i < numbers.length; i++) {
System.out.println(numbers[i]);
}
This code will print the value of each element in the numbers
array, one by one.
What are the different types of arrays in Java?
There are two main types of arrays in Java: single-dimensional arrays and multi-dimensional arrays.
Single-dimensional arrays
A single-dimensional array is an array that has only one dimension. For example, the numbers
array is a single-dimensional array.
An example is similar to what we discussed above.
int[] numbers = new int[10];
Multi-dimensional arrays
A multi-dimensional array is an array that has more than one dimension. For example, a 2D array is an array that has two dimensions.
2D Array
A 2D array is an array that has two dimensions. For example, a 2D array of integers could be used to store a table of data. The first dimension of the array would represent the rows in the table, and the second dimension would represent the columns in the table.
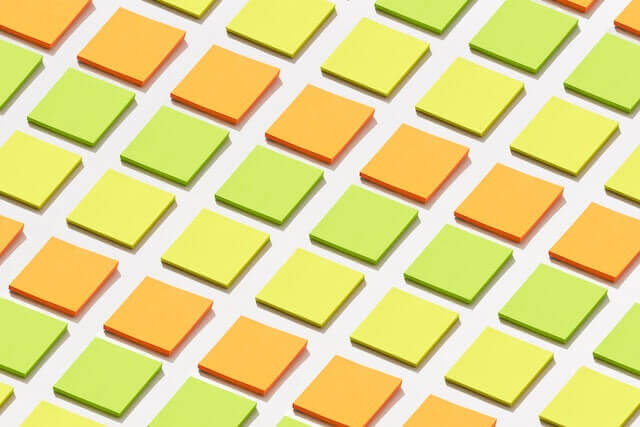
Here is an example of a 2D array of integers:
int[][] table = new int[3][4];
This array has 3 rows and 4 columns. The first row of the array would store the first 3 elements of the table, the second row would store the next 3 elements of the table, and so on.
3D Array
A 3D array is an array that has three dimensions. For example, a 3D array of integers could be used to store a volume of data. The first dimension of the array would represent the layers in the volume, the second dimension would represent the rows in the layer, and the third dimension would represent the columns in the layer.
Here is an example of a 3D array of integers:
int[][][] volume = new int[3][4][2];
This array has 3 layers, 4 rows, and 2 columns. The first layer of the array would store the first 3 * 4 * 2 elements of the volume, the second layer would store the next 3 * 4 * 2 elements of the volume, and so on.
Note that, to work on these multidimensional arrays, you need to think in terms of the dimensions you are adding.
What are the benefits of using arrays in Java?
There are several benefits to using arrays in Java. These include:
- Efficiency: Arrays are a very efficient way to store data in memory.
- Ease of use: Arrays are easy to use and understand.
- Flexibility: Arrays can be used to store a variety of data types.
Limitations to using arrays
- Fixed-size: Arrays have a fixed size. This means that you cannot add or remove elements from an array after it has been created.
- Complexity: Arrays can be complex to use in some cases.
- Memory usage: Arrays can use a lot of memory.
Overall, arrays are a powerful and versatile data structure in Java. They are efficient, easy to use, and flexible. However, they do have some limitations, such as their fixed size and potential memory usage.
Further Readings
- https://en.wikipedia.org/wiki/Array_(data_structure)
- Checkout our other blogs on other Data Structures
Feel free to share your thoughts on this topic in the comments section below 👇 We would be happy to hear and discuss the same 🙂