Annotations were introduced in Java SE 5, which was released in 2004. In Java, an annotation is a metadata that provides additional information about a program element. Annotations do not affect the execution of the program, but they can be used by the compiler and other tools to provide additional information about the program.
What are Annotations in Java?
Some of the Java inbuilt annotations are – @SuppressWarnings(“unchecked”), @Override, @Test, @Deprecated, etc.
Usage of Annotations
There are many different ways to use annotations in Java. Some common use cases include:
- Documentation: Annotations can be used to provide documentation for program elements. This documentation can be used by developers to understand the purpose of the program element and how it should be used.
- Code generation: Annotations can be used to generate code. This can be useful for tasks such as creating boilerplate code or generating code that is specific to a particular environment.
- Runtime processing: Annotations can be processed at runtime. This can be used to control the behavior of the program or to provide information to the user.
Since annotations are mostly metadata, you will see frameworks make the most use of it. For example, in earlier spring framework, there used to be lots of configurations being maintained in XML files which has mostly shifted to the annotations nowadays in the upgraded versions. In short, it makes the developers’ life very easy when it comes to code maintainability.
How to Create a Custom Annotation
To create a custom annotation in Java, you use the @interface
keyword. The “@interface
” keyword is followed by the name of the annotation. The annotation can have any number of methods. The methods in the annotation can have parameters, but the parameters cannot be primitive types. The methods in the annotation can have default values.
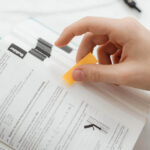
In Java, an annotation is a metadata that provides additional information about a program element
Here is an example of a custom annotation:
@interface Author {
String name() default "Unknown";
String email() default "Unknown";
}
This annotation has two methods: name()
and email()
. The “name()
” method has a default value of “Unknown”. The “email()
” method has a default value of “Unknown”.
How to use a custom annotation
To use a custom annotation, you use the @
symbol followed by the name of the annotation. The annotation can be applied to any program element, such as a class, method, or field. It has to be written in its own single line of code.
Here is an example of how to use the Author
annotation:
public class MyClass {
@Author(name = "John Doe", email = "[email protected]")
public static void main(String[] args) {
// Do something
}
}
In this example, the Author
annotation is applied to the main()
method. The name()
and email()
methods are used to specify the author of the main()
method.
How to access annotation value in the method
Even though, that’s not the intended use of annotations, you can still access the value of annotations in the respective method. For that, you need to do a little bit of modification in the custom annotations itself. Before that, let us learn about the meta annotations called Retention and Target which are two of the most important annotation attributes. They determine how and where an annotation is stored and used.
Retention
It specifies the time period during which an annotation is retained. There are three possible values for the retention attribute:
- SOURCE: The annotation is retained only in the source code. It is not stored in the compiled class file.
- CLASS: The annotation is retained in the compiled class file. It can be accessed by tools, such as IDEs and code generators.
- RUNTIME: The annotation is retained at runtime. It can be accessed by the Java Virtual Machine (JVM) and by other runtime tools.
Target
Target specifies the types of program elements that an annotation can be applied to. There are a number of possible values for the target attribute, including:
- TYPE: The annotation can be applied to classes, interfaces, and enums.
- FIELD: The annotation can be applied to fields.
- METHOD: The annotation can be applied to methods.
- PARAMETER: The annotation can be applied to method parameters.
- CONSTRUCTOR: The annotation can be applied to constructors.
- LOCAL_VARIABLE: The annotation can be applied to local variables.
- PACKAGE: The annotation can be applied to packages.
@Retention(RetentionPolicy.RUNTIME) // Make this annotation accessible at runtime via reflection.
@Target({ElementType.METHOD, <<More targets separated by comma>>}) // This annotation can only be applied to class methods.
Coming back to our original question, how to access the value of annotation in the corresponding method, you can make use of reflection in Java.
So, to get the value of author we can write a code something like this –
for (Method method: MyClass.class.getMethods() {
Author author = method.getAnnotation(Author.class);
if (author != null){
System.out.println(author.name());
System.out.println(author.name());
}
}
Make sure to use @Retention(RetentionPolicy.RUNTIME) while creating the Author Annotation.
Further Readings
- https://docs.oracle.com/javase/tutorial/java/annotations/basics.html
- https://docs.oracle.com/javase/8/docs/technotes/guides/language/annotations.html
- https://en.wikipedia.org/wiki/Java_annotation
- Checkout our other blogs on Core Java and Java Interview Questions
Annotations are a powerful tool that can be used to provide additional information about a program. Annotations can be used to improve the documentation of a program, to generate code, or to control the behavior of a program.
Feel free to share your thoughts on this topic in the comments section below 👇 We would be happy to hear and discuss the same 🙂
3 thoughts on “Java Annotations: A Practical Guide for Beginners”
I was trying to understand this topic from long time. Thanks for writing this. Can you please explain one more example for target meta annotation?
Hey There, Thanks for liking our post. 🙂
Coming to your question, actually it’s quite simple to understand. While retention meta annotation marks that the class or method or variable can be accessed based on the retention policy e.g. SOURCE, CLASS or RUNTIME, the same way you can setup/restrict the annotation to be used in certain part of the class using the “Target” Meta annotation. for example, if you mark an annotation with the ElementType.TYPE, it means that the said annotation can be applied only to classes, interfaces, and enums. If you use that annotation at some other place e.g. at a method, it will throw a compilation error. Something like – “The annotation @Author is disallowed for this location”
Hope this helps! 🙂
Great, thanks for the prompt response, I got the idea! I will try it out and let you know.