Yes, multithreading is a concurrency mechanism of Java. Concurrency is the ability of multiple threads to execute simultaneously. In Java, threads are lightweight processes that can run independently of each other.
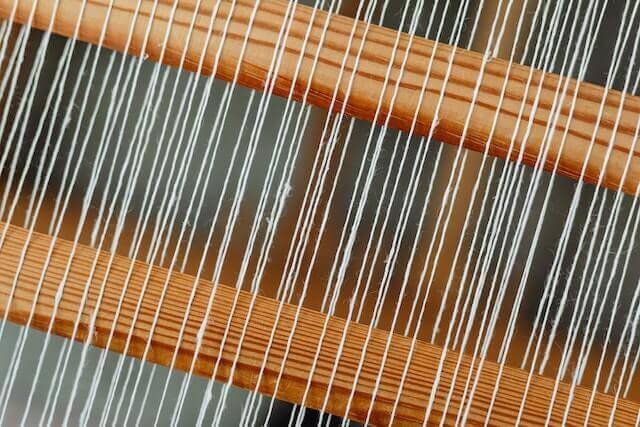
Here are some of the concurrency mechanisms available in Java:
- Thread: A thread is a lightweight process that can run independently of other threads.
- ExecutorService: An executor service is a pool of threads that can be used to execute tasks.
- Callable: A callable is a task that can be executed by an executor service.
- Future: A future is a reference to the result of a callable.
- Lock: A lock is a synchronization object that can be used to ensure that only one thread can access a shared resource at a time.
- Condition: A condition is a synchronization object that can be used to wait for a specific state to occur.
What is Multithreading
Multithreading is a programming concept that allows multiple tasks to be executed simultaneously. In Java, multithreading is supported through the Thread class. A thread is a lightweight process that can run independently of other threads.
Advantages & Disadvantages of Multithreading
There are many advantages to using multithreading. For example, it can improve the performance of an application by allowing multiple tasks to be executed at the same time. It can also make an application more responsive to user input.
However, there are also some disadvantages to using multithreading. For example, it can be more difficult to debug multithreaded applications. It can also be more difficult to ensure that data is accessed and modified safely in a multithreaded environment.
Multithreading in Java
Java supports multithreading through the Thread class. The Thread class provides methods for creating, starting, and stopping threads. It also provides methods for synchronizing threads and for pooling threads.
Example code
public class MultithreadedExample {
public static void main(String[] args) {
// Create two threads
Thread thread1 = new Thread(new Runnable() {
@Override
public void run() {
// Do something in thread 1
System.out.println("Thread 1 is running");
}
});
Thread thread2 = new Thread(new Runnable() {
@Override
public void run() {
// Do something in thread 2
System.out.println("Thread 2 is running");
}
});
// Start the threads
thread1.start();
thread2.start();
}
}
There are two ways to create a thread in Java:
- Extending the Thread class
- Implementing the Runnable interface
Extending the Thread class
To create a thread by extending the Thread class, you need to create a new class that inherits from the Thread class. The new class must override the run() method, which is the method that will be executed when the thread starts.
Here is an example of a thread that is created by extending the Thread class:
public class MyThread extends Thread {
@Override
public void run() {
// Do something in the thread
System.out.println("Thread 1 is running");
}
}
Implementing the Runnable interface
To create a thread by implementing the Runnable interface, you need to create a new class that implements the Runnable interface. The new class must have a run() method.
Here is an example of a thread that is created by implementing the Runnable interface:
public class MyRunnable implements Runnable {
@Override
public void run() {
// Do something in the thread
System.out.println("Thread 2 is running");
}
}
Once you have created a thread, you can start it by calling the start() method on the thread object.
Here is an example of how to start a thread in both classes.
public class ThreadExecutor{
public static void main(String []args){
MyRunnable t1 = new MyRunnable();
t1.run();
MyThread t2 = new MyThread();
t2.start();
}
}
When you start a thread, it will start executing the run() method. The run() method will continue to execute until it is finished.
Output:
Thread 1 is running
Thread 2 is running
It is important to note that threads are independent of each other. This means that they can run at the same time, and they can access and modify shared data. If two threads are accessing and modifying the same data at the same time, it can lead to race conditions.
Race conditions are a type of bug that can occur in multithreaded applications. They can be difficult to find and fix, so it is important to avoid them.
To avoid race conditions, you can use synchronization mechanisms, such as the synchronized keyword and the Lock class.
- The synchronized keyword can be used to lock a piece of data, preventing other threads from accessing the data until the lock is released.
- The Lock class provides a more flexible way to lock data. A Lock object can be used to lock a piece of data, and then other threads can wait for the lock to be released before they can access the data.
- By using synchronization mechanisms, you can help to ensure that your multithreaded applications are safe and reliable.
Synchronizing threads
When multiple threads are accessing the same data, it is important to synchronize access to the data to ensure that the data is accessed and modified safely. Java provides several methods for synchronizing threads, including the synchronized keyword and the Lock class.
The synchronized keyword can be used to synchronize access to a block of code. When a thread executes a block of code that is synchronized, other threads will not be able to execute the same block of code at the same time.
The Lock class provides a more flexible way to synchronize access to data. A Lock object can be used to lock a piece of data, preventing other threads from accessing the data until the lock is released.
Go through our other blog on Deadlocks: How to Avoid Them with Synchronization to gain a complete understanding of Synchronization concepts in multithreading.
Pooling threads
Thread pooling is a technique for reusing threads. When you use thread pooling, you create a pool of threads that are available to execute tasks. When a task needs to be executed, you request a thread from the pool. After the task is complete, the thread is returned to the pool.
Thread pooling can improve the performance of an application by reducing the number of times that threads need to be created and destroyed.
Examples of multithreading in Java
There are many examples of multithreading in Java. Some common examples include:
- Downloading files
- Parsing large data sets
- Rendering graphics
- Networking applications
- Load and stress testing
By using these concurrency mechanisms, developers can create Java applications that can take advantage of multiple CPUs and improve the performance and responsiveness of their applications.
Further Readings
- https://en.wikipedia.org/wiki/Java_concurrency
- Checkout our other blogs on Java Concepts
Feel free to share your thoughts on this topic in the comments section below 👇 We would be happy to hear and discuss the same 🙂