Primitive type data is passed by value and objects are passed by reference. But wait a minute, what happens when the references are passed in the method calls? In this article, we will figure out the Truth About Java’s Pass-by-Value Mechanism.
Is it Pass by reference or Pass by Value?
In Java, when object references are passed, they are also passed as value. Hence, in a nutshell, we can say that Java is always “pass by value“. It could be confusing for the newbies but take a good look and you will understand what it means. Let us check it with an example code –
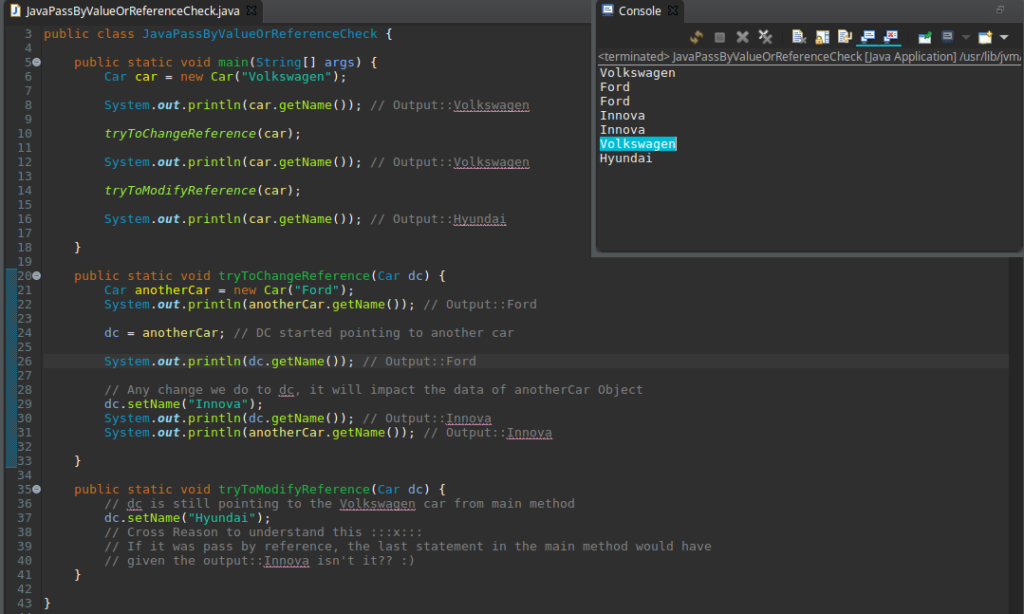
Sample Code
public class JavaPassByValueOrReferenceCheck {
public static void main(String[] args) {
Car car = new Car("Volkswagen");
System.out.println(car.getName()); // Output::Volkswagen
tryToChangeReference(car);
System.out.println(car.getName()); // Output::Volkswagen
tryToModifyReference(car);
System.out.println(car.getName()); // Output::Hyundai
}
public static void tryToChangeReference(Car dc) {
Car anotherCar = new Car("Ford");
System.out.println(anotherCar.getName()); // Output::Ford
dc = anotherCar; // DC started pointing to another car
System.out.println(dc.getName()); // Output::Ford
// Any change we do to dc, it will impact the data of anotherCar Object
dc.setName("Innova");
System.out.println(dc.getName()); // Output::Innova
System.out.println(anotherCar.getName()); // Output::Innova
}
public static void tryToModifyReference(Car dc) {
// dc is still pointing to the Volkswagen car from main method
dc.setName("Hyundai");
// Cross Reason to understand this :::x:::
// If it was pass by reference, the last statement in the main method would have
// given the output::Innova isn't it?? :)
}
}
class Car {
private String name;
public Car(String name) {
this.name = name;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
Try to run the above code in debug mode and you will see how it works. Also, you may comment or un-comment on the main method and see the different scenarios
Explanation Video
Still confused? Watch this Debug video.
Further Readings
- https://community.oracle.com/tech/developers/discussion/2110675/pass-by-reference-or-pass-by-value
- https://stackoverflow.com/a/40523
- http://www.javadude.com/articles/passbyvalue.htm
- https://community.oracle.com/tech/developers/discussion/comment/9034101#Comment_9034101
If you still have doubts and want to discuss, feel free to share your viewpoints in the comment section below. 🙂
One thought on “The Truth About Java’s Pass-by-Value Mechanism”
Superb! I liked the blog because you have explained it with example. Good work (y)