Java Generics are a powerful feature of Java that can help you to write more robust and efficient code. They can be used to prevent errors at compile time, to improve performance, and to make code more reusable.
What are Java Generics?
Java Generics are a feature of the Java programming language that allows you to specify the type of data that can be stored in a class, method, or variable. This helps to prevent errors and improve performance.
For example, a generic class called List can be used to store any type of data, such as Integer, String, or Customer. This is in contrast to a non-generic class called ArrayList, which can only store objects of type Object.
Why use Java Generics?
Generics are a powerful feature of Java that can help you to write more robust and efficient code. It can provide type safety to your code. Increases code reusability and readability. It also improves the performance and maintainability of your code.
How do Java Generics work?
Generics in Java work by using type parameters. A type parameter is a placeholder for a type. When you declare a generic class, method, or variable, you specify the type parameters that it uses.
For example, the following code declares a generic class called List that uses a type parameter called T:
class List<T> {
private T[] elements;
public List() {
elements = new T[0];
}
public void add(T element) {
elements.add(element);
}
public T get(int index) {
return elements[index];
}
}
This class can be used to store any type of data, as long as it is compatible with the type parameter T. For example, you could create a list of Integer objects, a list of String objects, or a list of any other type of object.
When you use a generic class, method, or variable, the compiler will automatically insert the type parameters that you specified when you declared it. For example, the following code creates a list of Integer objects:
List<Integer> list = new List<>();
list.add(10);
int value = list.get(0);
The compiler will insert the type parameter Integer for T when it compiles this code. This ensures that the compiler can perform type-checking and that the code will run correctly.
Here are some additional details about how generics work:
Type erasure
When a generic class or method is compiled, the type parameters are erased. This means that the compiler generates code that does not contain any references to the type parameters. This is done to improve performance and compatibility.
Wildcards
Wildcards can be used to specify that a generic type can be used with any type, or with a specific type or type. For example, the following code declares a method that takes a list of any type.
public void method(List<?> list) {
// ...
}
This method can be used with any type of list, such as a list of Integer objects, a list of String objects, or a list of any other type of object.
Parameterized types
Parameterized types can be used to create a specific instance of a generic type. For example, the following code creates a list of Integer objects:
List<Integer> list = new ArrayList<>();
This creates a list of Integer objects that is an instance of the generic class List.
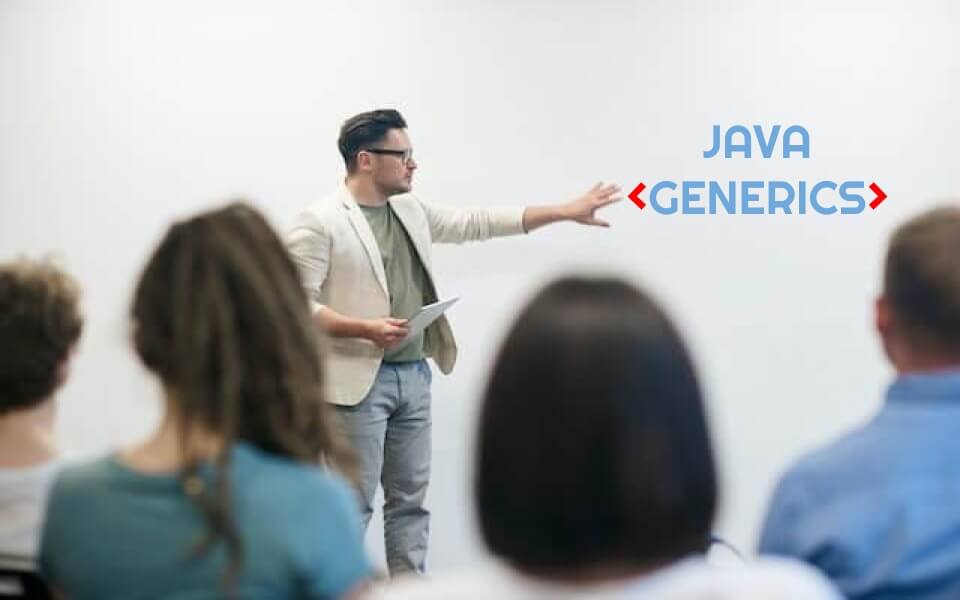
Examples of using Java Generics
Java Generics classes
Generic classes are declared using the <>
symbol. The type parameter(s) can be any non-primitive type, such as String
, Integer
, or List
. For example, the following code declares a generic class called Box
that can store any type of object:
public class Box<T> {
private T item;
public void setItem(T item) {
this.item = item;
}
public T getItem() {
return item;
}
}
Once a generic class is declared, it can be used to create instances of that class that can store objects of the specified type. For example, the following code creates an instance of Box that can store a String object:
Box<String> myBox = new Box<>();
myBox.setItem("Hello, world!");
String item = myBox.getItem();
Generic classes can also be used to declare methods. The type parameter(s) can be used in the method declaration, the method body, and the method return type. For example, the following code declares a generic method called printItem() that can print any type of object:
public <T> void printItem(T item) {
System.out.println(item);
}
This method can be used to print objects of any type. For example, the following code prints a String object:
printItem("Hello, world!");
Java Generics methods
Java generics methods are methods that can be used with any type of data. This is done by using type parameters in the method declaration. Type parameters are placeholders for the actual type of data that will be used with the method.
For example, the following code declares a generic method called swap that can swap the values of two variables:
public <T> void swap(T a, T b) {
T temp = a;
a = b;
b = temp;
}
This method can be used to swap the values of any type of data. For example, the following code swaps the values of two String objects:
String a = "Hello";
String b = "World";
swap(a, b);
System.out.println(a); // World
System.out.println(b); // Hello
Here are some additional things to keep in mind when using generics methods:
Type parameters can be bounded: Type parameters can be bounded to restrict the types of data that can be used with the method. For example, the following code declares a generic method called max that can find the maximum value of two numbers:
public <T extends Number> T max(T a, T b) {
if (a.compareTo(b) > 0) {
return a;
} else {
return b;
}
}
This method can only be used with numbers because the type parameter T is bounded to the Number class.
Type parameters can be wildcards: Type parameters can also be used as wildcards. A wildcard is a placeholder for any type. For example, the following code declares a generic method called print that can print the value of any object:
public <T> void print(T object) {
System.out.println(object);
}
This method can be used to print objects of any type because the type parameter T is a wildcard.
Java Generics collections
Java generics collections are collections that can store objects of any type. This is done by using type parameters in the collection declaration. Type parameters are placeholders for the actual type of data that will be stored in the collection.
For example, the following code declares a generic collection called List that can store any type of object:
public class List<T> {
private T[] items;
public void add(T item) {
items[items.length] = item;
}
public T get(int index) {
return items[index];
}
}
This collection can be used to store objects of any type. For example, the following code stores a String object in the collection:
List<String> myList = new List<>();
myList.add("Hello, world!");
String item = myList.get(0);
Here are some additional things to keep in mind when using generics collections:
Type parameters can be bounded: Type parameters can be bounded to restrict the types of data that can be stored in the collection. For example, the following code declares a generic collection called List that can only store numbers:
public class List<T extends Number> {
private T[] items;
public void add(T item) {
items[items.length] = item;
}
public T get(int index) {
return items[index];
}
}
This collection can only be used to store numbers because the type parameter T is bounded to the Number class.
Type parameters can be wildcards: Type parameters can also be used as wildcards. A wildcard is a placeholder for any type. For example, the following code declares a generic collection called List that can store objects of any type:
public class List<T> {
private T[] items;
public void add(T item) {
items[items.length] = item;
}
public T get(int index) {
return items[index];
}
}
This collection can be used to store objects of any type because the type parameter T is a wildcard.
Benefits of using Java Generics
Here are some of the benefits of using generics:
- Type safety: Generics help to prevent errors at compile time by ensuring that the types of data used in a class, method, or variable are compatible. This can help to prevent runtime errors such as ClassCastException.
- Code reusability: Generics can be used to create reusable code that can be used with different types of data.
- Readability: Generics make code more readable by making it clear what type of data is being stored in a class or method. This can help to avoid errors and make code easier to maintain.
- Improved performance: Generics can improve performance by eliminating the need for type casts and by allowing the compiler to perform optimizations.
- Maintainability: Generics can make code more maintainable by reducing the need for casts and other types of type checking. This can make code easier to read and understand, and it can also make it easier to find and fix errors.
Drawbacks of using Java Generics
While there are benefits of Java Generics, Here are some of the drawbacks of using Java generics:
- Performance: Generics can add some overhead to the execution of Java programs. This is because generics are implemented using type erasure, which means that the compiler removes all references to type parameters at runtime. This can lead to a small performance penalty, but it is usually not significant.
- Type safety: Generics cannot guarantee type safety in all cases. For example, if a generic method is called with an argument of the wrong type, a ClassCastException will be thrown at runtime. This can be avoided by using type assertions, but it can be difficult to remember to use them in all cases.
- Code complexity: Generics can make code more complex, especially when dealing with generics and inheritance. This is because generics can introduce new types that are not present in the underlying Java language. This can make it difficult to understand and maintain code that uses generics.
Overall, the drawbacks of using Java generics are relatively minor. The benefits of using generics, such as type safety and readability, far outweigh the drawbacks.
Recommendations for when to use Java Generics
Here are some recommendations for when to use Java generics:
- Use generics when working with collections. Generics can be used to ensure that only objects of the correct type can be stored in a collection. This can help to prevent runtime errors such as
ClassCastException
. - Use generics when writing generic methods. Generic methods can be used to work with objects of any type. This can make code more reusable and easier to read.
- Use generics when working with generic types. Generic types can be used to create new types that are specific to a particular use case. This can make code more concise and easier to understand.
Here are some examples of when not to use Java generics:
- Do not use generics when performance is critical. Generics can add some overhead to the execution of Java programs. If performance is critical, it may be better to use non-generic code.
- Do not use generics when you need to use reflection. Reflection can bypass the type safety of generics. If you need to use reflection, it may be better to use non-generic code.
- Do not use generics when you need to work with primitive types. Primitive types cannot be used with generics. If you need to work with primitive types, it may be better to use non-generic code.
Overall, generics are a powerful feature that can be used to improve the safety, readability, and maintainability of Java code. However, it is important to use generics wisely and to avoid using them in situations where they may not be appropriate.
Feel free to share your thoughts on this topic in the comments section below 👇 We would be happy to hear and discuss the same 🙂
Search Keywords of this topic: Java Generics tutorial, Java generics examples, Java generics vs. non-generics, How to use Java generics, What are Java generics?, Java generics wildcards, Java generics collections, Java generics constraints, Java generics type erasure, Java generics performance