The synchronized keyword is used to control access to shared resources in Java. When a block of code is synchronized, only one thread can execute that block of code at a time. This prevents race conditions, which can occur when multiple threads try to access the same resource at the same time. Deadlocks can occur when two or more threads are waiting for each other to release a lock. Go through our other blog on Multithreading: How to Implement Java Concurrency? to understand the basics of multithreading if you are not aware of it.
What is the use of the “synchronized” keyword in Java?
The synchronized keyword can be used on methods and blocks of code. When synchronized is used on a method, the lock is acquired on the object that the method is called on. When synchronized is used on a block of code, the lock is acquired on the object that is specified in the parentheses after the synchronized keyword.
The synchronized keyword can be used to improve performance in multithreaded applications. By preventing race conditions, the synchronized keyword can ensure that shared resources are accessed in a consistent manner. This can reduce the amount of time that threads spend waiting for access to shared resources, which can improve the overall performance of the application.
Here are some examples of how the synchronized keyword can be used:
public synchronized void method() {
// Code that accesses shared resources
}
public static synchronized void staticMethod() {
// Code that accesses shared resources
}
synchronized (object) {
// Code that accesses shared resources
}
The synchronized keyword is a powerful tool that can be used to improve the performance and safety of multithreaded applications. However, it is important to use the synchronized keyword wisely. If used too often, it can lead to performance problems. It is also important to note that the synchronized keyword does not prevent deadlocks. Deadlocks can occur when two or more threads are waiting for each other to release a lock.
How can the “synchronized” keyword improve performance?
the synchronized keyword can improve performance in multithreaded applications. By preventing race conditions, the synchronized keyword can ensure that shared resources are accessed in a consistent manner. This can reduce the amount of time that threads spend waiting for access to shared resources, which can improve the overall performance of the application.
However, it is important to note that the synchronized keyword can also introduce some performance overhead. This is because when a thread acquires a lock, it must block other threads from accessing the same resource. This can lead to increased context switching and decreased throughput.
The impact of the synchronized keyword on performance will depend on a number of factors, including the number of threads in the application, the number of shared resources, and the frequency with which the shared resources are accessed. In general, the synchronized keyword will have a more significant impact on performance in applications with a large number of threads and a high frequency of shared resource access.
In some cases, the use of the synchronized keyword may actually degrade performance. This can happen if the synchronized block is too large or if the shared resource is not accessed frequently. In these cases, it may be better to use other synchronization mechanisms, such as volatile variables or concurrent collections.
Can the synchronized keyword not prevent Deadlocks?
The synchronized keyword does not prevent deadlocks. Deadlocks can occur when two or more threads are waiting for each other to release a lock.
Here is an example of how a deadlock can occur with the synchronized keyword:
class Deadlock {
private Object lock1 = new Object();
private Object lock2 = new Object();
public void method1() {
synchronized (lock1) {
System.out.println("Thread 1 acquired lock 1");
synchronized (lock2) {
System.out.println("Thread 1 acquired lock 2");
}
}
}
public void method2() {
synchronized (lock2) {
System.out.println("Thread 2 acquired lock 2");
synchronized (lock1) {
System.out.println("Thread 2 acquired lock 1");
}
}
}
}
In this example, thread 1 calls method1(), which acquires lock1 and then waits to acquire lock2. Thread 2 calls method2(), which acquires lock2 and then waits to acquire lock1. As a result, thread 1 is waiting for thread 2 to release lock2, and thread 2 is waiting for thread 1 to release lock1. This is a deadlock, and neither thread will be able to continue execution.
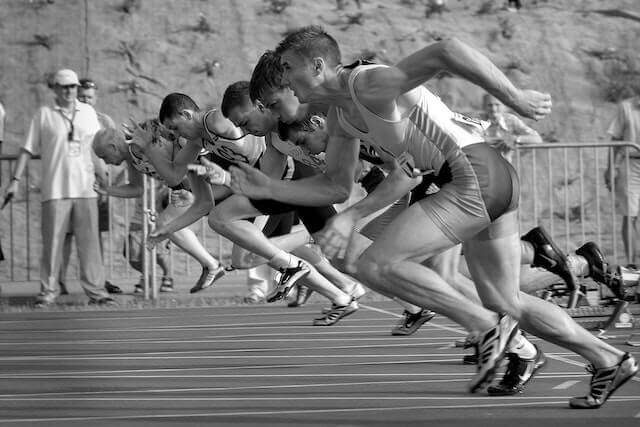
To prevent deadlocks, it is important to use the synchronized keyword carefully. You should avoid using the synchronized keyword on multiple objects if possible. If you do need to use the synchronized keyword on multiple objects, you should make sure that the threads acquire the locks in the same order. You can also use other synchronization mechanisms, such as semaphores or barriers, to help prevent deadlocks.
Further Readings
- https://en.wikipedia.org/wiki/Synchronization_(computer_science)
- Check out other blogs on Java Concepts and Java Interview Questions
Feel free to share your thoughts on this topic in the comments section below 👇 We would be happy to hear and discuss the same 🙂